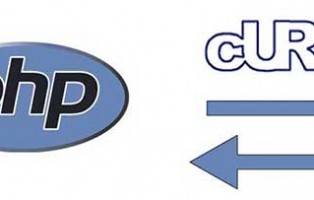
This is an easy to use PHP/cURL class to handle most needed tasks
Table of Contents
Installation
To download file
Post Form
GET form
Retrieve Header
basic authentication
Returned Data
Options
Download
How to examples
Installation
To utilize this class, first import curl.class.php into your project, and require it.
require_once ('curl.class.php');
Download file
$curl = new Curl();
$curl->getFile('http://www.example.com');
$curl->file; // this will contain the fetched file
Post Form
include 'curl.class.php';
$curl = new Curl();
$data_array['code'] = '55445';
$data_array['session'] = time();
$data_array['zipcode'] = '52415';
$data_array['Submit'] = 'Submit';
$curl->postForm('http://www.webbotsspidersscreenscrapers.com/zip_code_form.php', $data_array);
so the $data_array key is the form field name and array value is set to the field value. For examples. $data_array[‘zipcode’] = ‘52415’; . [‘zipcode’]=> form field name ‘52415’=> form field value .
Retrieve Header
include 'curl.class.php';
$curl = new Curl();
$curl->getHeader('http://www.example.com/');
in this case the request method is HEAD and no body is returned
Get form
include 'curl.class.php';
$curl = new Curl();
$data_array['term'] = 'games';
$data_array['sort'] = 'up';
$curl->getForm('http://www.webbotsspidersscreenscrapers.com/search/search.php', $data_array);
Submits a form with the GET method
Basic authentication
include 'curl.class.php';
$curl = new Curl();
$username = 'webbot';
$password = 'sp1der3';
$curl->basicAuth($username, $password);
$curl->getFile('http://www.webbotsspidersscreenscrapers.com/basic_authentication');
To login using basic authentication
Returned Data
All of these examples will return 3 results
1- Contents of the fetched file
$curl->file
2- Get information regarding a specific transfer
$curl->infoArray
it returns a lot of useful information as an associative array
3- return the last error generated by cURL
$curl->error
Options
change agent name
the agent name to use instead of the default ( the default is set to Firefox )
$curl->setAgent('your agent name')
Time out
how long to wait for a response from a server instead of the default ( the default is 25 seconds )
$curl->setTimeOut()
Cookie location
Location of your cookie file instead of the default
( the default is cookie.txt ) to the script home directory )
$curl->setCookieFile()
the path you specify must be relative for example if you have a folder named tmp and want the cookie to saved to this folder it will be like that
example
$curl->setCookieFile('tmp/cookie.txt')
referer
the referer to use instead of the default (the default is https://www.google.com)
$curl->setReferer()
Include header
to include response header with the body
$curl->includeHeader()
Verbose
To enable verbose which will write more details about the transfer
$curl->enableVerbose()
To change the location of your Verbose file (the default is verbose.txt resides in the script home directory)
the path you specify must be relative for example
if you have a folder named tmp and want the verbose file to saved to this folder it will be like that
example
$curl->setVerboseFile('tmp/cookie.txt')
Redirection limits
To change the max redirections (the default is 4)
$curl->setMaxRedirections ($max)
An array of HTTP header fields to set
$curl->addHeader ()
example
$header_array[] = "Accept-Encoding: compress, gzip";
Note that it expects to receive data in an array
Download
to download from php cURL class github